Angular에서 Excel Spreadsheets 가져오고/내보내기
페이지 정보
작성자 GrapeCity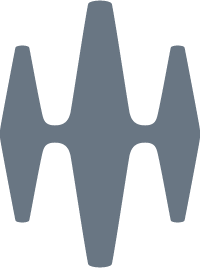
본문
첨부파일
관련링크
Excel 스프레드 시트는 1980 년대부터 사용되었습니다. 3천만 명이 넘는 사용자는 대부분 Excel 스프레드 시트 환경에 익숙합니다. 많은 비즈니스에서 예산 및 계획을 위해 Excel 스프레드 시트를 사용하고 있습니다. 하지만, 조직이 성장함에 따라 Excel의 기능에 의존하기가 어렵다는 것을 알게 될 것입니다.
응용 프로그램에서 스프레드시트 구성 요소를 사용할 때의 이점
스프레드 시트 구성 요소는 향상된 보안, 데이터 통합, 향상된 데이터 시각화, 전략적 성능 측정 (SPM), 정교한 통계 분석 등을 제공 할 수 있습니다.
JavaScript 컴포넌트인 SpreadJS 는 친숙한 Excel 스프레드 시트 인터페이스를 제공합니다. Excel 파일을 가져오고 내보낼 수 있으며, Excel에 의존하지 않고도 JavaScript로 성과 및 비즈니스 대시 보드를 작성할 수 있습니다.
Angular 지원을 통해이 문서에서는 Angular 환경에서 SpreadJS를 사용하여 Excel 스프레드 시트를 가져오고 내보내는 방법을 보여줍니다.
먼저 앱에 SpreadJS 구성 요소를 설치하십시오.
SpreadJS의 Excel 가져 오기 및 내보내기 기능을 사용하므로 ExcelIO 구성 요소가 필요합니다. npm을 사용하여 설치할 수 있습니다.
Angular에서 Excel 스프레드 시트를 가져오고 내보내는 방법
SpreadJS는 다음과 같이 html 페이지에 추가 할 수 있습니다.
<gc-spread-sheets [hostStyle]="hostStyle" (workbookInitialized)="workbookInit($event)">
</gc-spread-sheets>
SpreadJS 컴포넌트를 인스턴스화하고, 다음 코드를 사용하여 app.component.ts 파일 에서 ExcelIO 클래스의 오브젝트를 작성 하십시오.
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
spreadBackColor = 'aliceblue';
hostStyle = {
width: '95vw',
height: '80vh'
};
private spread: GC.Spread.Sheets.Workbook;
private excelIO;
constructor() {
this.excelIO = new Excel.IO();
}
workbookInit(args) {
const self = this;
self.spread = args.spread;
const sheet = self.spread.getActiveSheet();
sheet.getCell(0, 0).text('Test Excel').foreColor('blue');
sheet.getCell(1, 0).text('Test Excel').foreColor('blue');
sheet.getCell(2, 0).text('Test Excel').foreColor('blue');
sheet.getCell(3, 0).text('Test Excel').foreColor('blue');
sheet.getCell(0, 1).text('Test Excel').foreColor('blue');
sheet.getCell(1, 1).text('Test Excel').foreColor('blue');
sheet.getCell(2, 1).text('Test Excel').foreColor('blue');
sheet.getCell(3, 1).text('Test Excel').foreColor('blue');
sheet.getCell(0, 2).text('Test Excel').foreColor('blue');
sheet.getCell(1, 2).text('Test Excel').foreColor('blue');
sheet.getCell(2, 2).text('Test Excel').foreColor('blue');
sheet.getCell(3, 2).text('Test Excel').foreColor('blue');
sheet.getCell(0, 3).text('Test Excel').foreColor('blue');
sheet.getCell(1, 3).text('Test Excel').foreColor('blue');
sheet.getCell(2, 3).text('Test Excel').foreColor('blue');
sheet.getCell(3, 3).text('Test Excel').foreColor('blue');
}
Export(가져오기)를 위해 xlsx 파일을 허용하는 입력 요소를 만듭니다.
app.component.html에 다음 코드를 추가해 봅시다 :
<div class='loadExcelInput'>
<p>Open Excel File</p>
<input type="file" name="files[]" multiple id="jsonFile" accept=".xlsx" (change)="onFileChange($event)" />
</div>
ExcelIO 객체는 선택된 파일을 열 수 있으며, 결과는 json 입니다.
이 JSON 데이터는 SpreadJS에서 직접 이해할 수 있습니다. 따라서 아래와 같이 change 이벤트에 대한 onFileChange () 함수에서 Import(가져오기) 코드를 작성 합니다.
onFileChange(args) {
const self = this, file = args.srcElement && args.srcElement.files && args.srcElement.files[0];
if (self.spread && file) {
self.excelIO.open(file, (json) => {
self.spread.fromJSON(json, {});
setTimeout(() => {
alert('load successfully');
}, 0);
}, (error) => {
alert('load fail');
});
}
}
마찬가지로 Export(내보내기) 기능을 처리하는 버튼을 추가하겠습니다. 내보내기 버튼을 추가하기 위해 다음을 사용할 수 있습니다.
<div class='exportExcel'>
<p>Save Excel File</p>
<button (click)="onClickMe($event)">Save Excel!</button>
</div>
이 버튼의 클릭 이벤트를 처리하고 코드를 작성해야합니다. SpreadJS는 데이터를 JSON으로 저장하고 해당 JSON을 ExcelIO에서 사용하여 BLOB으로 저장할 수 있습니다. 나중에, 이 BLOB데이터는 다른 구성 요소 ( file-saver )를 사용하여 주어진 형식에 따라 저장해야합니다 .
onClickMe(args) {
const self = this;
const filename = 'exportExcel.xlsx';
const json = JSON.stringify(self.spread.toJSON());
self.excelIO.save(json, function (blob) {
saveAs(blob, filename);
}, function (e) {
console.log(e);
});
}
내보내기 기능을 달성하기 위해 file-saver 컴포넌트 사용했습니다. 프로젝트에 file-saver를 포함 시키려면 다음 단계를 따르십시오.
2. 이 타사 라이브러리를 '.angular.json'에 추가하십시오.
"scripts": ["./node_modules/file-saver/FileSaver.js"]**
3. 구성 요소 가져 오기
import {saveAs} from 'file-saver';
댓글목록
등록된 댓글이 없습니다.