Winforms윈폼 WinForms Datagrid의 사용자 정의 데이터 필터를 만드는 방법
페이지 정보
작성자 GrapeCity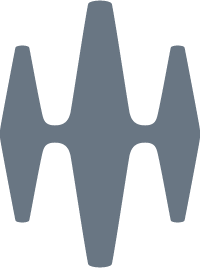
본문
관련링크
이 기능은 이미 IC1ColumnFilter 및 IC1ColumnFilterEditor 인터페이스를 사용하여 FlexGrid 컨트롤의 라이브러리에 내장되어 있습니다. 이번 스터디에서는 .NET 6 응용 프로그램에서 FlexGrid DateTime 열에 "마지막 회계 연도"와 같은 사용자 정의 필터를 적용하는 방법에 대해 알아보겠습니다.
프로젝트 설정
먼저 Visual Studio를 열고 새 .NET 6 WinForms 응용 프로그램을 만듭니다. 이름은 "FlexGridCustomFilters"로 지정하겠습니다. 여러분은 스터디 마지막에서 해당 프로젝트 다운로드 링크를 확인할 수 있습니다.
다음으로, NuGet 패키지 관리자를 통해 C1.Win.FlexGrid 6.0 .dll 파일을 프로젝트에 다운로드합니다. 설치에 성공하면 FlexGrid를 폼에 드래그 앤 드롭 할 수 있습니다. 일부 Dummy 전송 데이터를 DataTable 개체에 추가한 다음, FlexGrid에 바인딩합니다. Dummy 전송 데이터는 800 일 전으로 되돌아가 매일 사용자 정의 날짜 필터링을 테스트할 수 있습니다.
사용자 정의 필터 만들기
이제 데이터가 채워진 FlexGrid가 있으므로, "배송 날짜" 열의 사용자 정의 필터를 생성해 보겠습니다. 참고로, "배송 날짜" 열에는 800 일 전 또는 2 년 전이 넘는 과거로 돌아가는 DateTime 값이 포함됩니다.
이 샘플의 경우, 아래 스크린샷과 유사하게 런타임에서 필터를 선택할 때 적합한 체크박스를 선택하면 그리드를 다시 로드하여 "지난 주", "이번 회계 연도" 또는 "지난 회계 연도" 주문만 표시할 수 있도록 사용자 정의 필터를 생성하라는 지시를 받았다고 가정하겠습니다.
사용자 정의 필터를 만들려면 를 구현하는 필터 클래스와 를 구현하는 편집기 클래스를 생성해야 합니다. 편집기를 사용하여 런타임에 필터를 수정하고 구성할 수 있습니다.
IC1ColumnFilter로 필터 클래스 만들기
먼저, IC1ColumnFilter 인터페이스를 구현하는 필터 클래스부터 시작해 보겠습니다. 프로젝트에 DateFilter.cs라는 새 파일을 만들고 클래스를 선언하는 동안 다음과 같이 작성하여 IC1ColumnFilter 인터페이스를 구현하겠습니다.
class DateFilter : C1.Win.FlexGrid.IC1ColumnFilter
런타임에 DateFilter 값을 추적하기 위한 몇 가지 필드를 만듭니다.
DateTime _min = DateTime.MinValue; DateTime _max = DateTime.MaxValue;
그런 다음 필터의 최소값 및 최대값에 대해 개체 모델의 게터 & 세터 (getter and setter) 메서드에 추가합니다.
/// <summary> /// Gets or sets the minimum date required by the filter. /// </summary> public DateTime Minimum { get { return _min; } set { _min = value; } } /// <summary> /// Gets or sets the maximum date required by the filter. /// </summary> public DateTime Maximum { get { return _max; } set { _max = value; } }
마지막으로, IsActive 속성인 IC1ColumnFilter와 Reset(), Apply(), 및 GetEditor() 메서드에서 구현하는 필수 멤버를 포함해야 합니다.
#region ** IC1ColumnFilter Members // filter is active if range doesn't cover all represetable dates. public bool IsActive { get { return _min != DateTime.MinValue || _max != DateTime.MaxValue; } } // reset filter. public void Reset() { _min = DateTime.MinValue; _max = DateTime.MaxValue; } // apply filter to a given date public bool Apply(object value) { var dt = (DateTime)value; return dt >= _min && dt <= _max; } // return editor control for this filter IC1ColumnFilterEditor IC1ColumnFilter.GetEditor() { return new DateFilterEditor(); } #endregion
IC1ColumnFilterEditor로 필터 편집기 만들기
다음으로, IC1ColumnFilterEditor 인터페이스를 구현하는 편집기 클래스를 포함할 새 사용자 컨트롤을 프로젝트에 추가해야 합니다.
이 사용자 컨트롤의 이름을 DateFilterEditor.cs로 설정하고 다음과 같이 공개 부분 클래스를 정의하겠습니다.
public partial class DateFilterEditor : UserControl, C1.Win.FlexGrid.IC1ColumnFilterEditor
먼저 일부 표준 체크박스에 추가할 사용자 컨트롤의 디자이너 보기로 이동해야 합니다. DateFilterEditor 클래스에 포함될 사용자 정의 필터 메서드 내에서 활용하겠습니다.
"지난 주", "이번 회계 연도" 및 "마지막 회계 연도"에 대한 결과를 보려면 세 개의 체크박스를 사용자 컨트롤에 끌어다 놓습니다. 그리고 이에 따라 이름을 설정해야 합니다. 해당 스터디 후반부에서 이 체크박스를 선택한 경우 발생하는 로직을 설정할 수 있습니다.
이전에 만든 DateFilter 클래스와 유사하게 다음과 같은 필드 및 구성자에 대한 영역이 있습니다.
#region ** fields DateFilter _filter; #endregion //------------------------------------------------------------------------------- #region ** ctor public DateFilterEditor() { InitializeComponent(); } #endregion
IC1ColumnFilter를 구현한 DateFilter와 마찬가지로 DaterFilterEditor 클래스의 경우 DateFilterEditor 개체가 인터페이스 구현으로 다형성을 통해 IC1ColumnFilterEditor 유형을 상속하도록 IC1ColumnFilterEditor를 구현해야 합니다.
IC1ColumnFilterEditor 인터페이스를 구현하는 동안 KeepFormOpen 속성과 Initialize() 및 ApplyChanges() 메서드를 만들어야 합니다. ApplyChanges() 메서드는 사용자 정의 필터 선택 항목에 로직을 적용할 위치입니다. 다음은 사용자 정의 필터 설정을 완료하는 것입니다.
#region ** IC1ColumnFilterEditor public void Initialize(C1.Win.FlexGrid.C1FlexGridBase grid, int columnIndex, C1.Win.FlexGrid.IC1ColumnFilter filter) { _filter = (DateFilter)filter; } public void ApplyChanges() { // Begin ApplyChanges() method by resetting the filter states when a new filter is applied. _filter.Reset(); // Set filter rules for last week filter if (_chkLastWeek.Checked) { _filter.Maximum = DateTime.Today.AddDays(-7); // This while loop will EXCLUDE Saturday from the result while (_filter.Maximum.DayOfWeek != DayOfWeek.Saturday) { _filter.Maximum = _filter.Maximum.AddDays(1); } _filter.Minimum = DateTime.Today.AddDays(-7); // This while loop will INCLUDE Monday from the result. while (_filter.Minimum.DayOfWeek != DayOfWeek.Monday) { _filter.Minimum = _filter.Minimum.AddDays(-1); } } // Set filter rules for last Fiscal Year. In this case, we want to say that the Fiscal Year starts April 1st and ends March 31st. if (_chkLastFiscalYear.Checked) { _filter.Maximum = DateTime.Today.AddYears(-1); _filter.Minimum = DateTime.Today.AddYears(-1); // Set maximum filter to end at March 31st of current year. while (_filter.Maximum.Month != 4 || _filter.Maximum.Day != 1) { _filter.Maximum = _filter.Maximum.AddDays(1); } // Set minimum filter to start at April 1st of last year. while (_filter.Minimum.Month != 4 || _filter.Minimum.Day != 1) { _filter.Minimum = _filter.Minimum.AddDays(-1); } } // Set the filter rules for this Fiscal Year. In this case, we want to say everything from the most recent April 1st up the present. Thus, we need two internal if statements to determine if April has already passed for this year. if(_chkThisFiscalYear.Checked) { // Set the maximum to the current day + 1, since Maximum Bound for the filter is exclusive. _filter.Maximum = DateTime.Today.AddDays(1); // If today's month is January through March, then subtract a year from the minimum. For example, if today is January 2023, that means Fiscal Year started April 2022, so we have to use the AddYears(-1).Year when setting the DateTime for the minimum filter value. if(DateTime.Today.Month < 4) { _filter.Minimum = new DateTime(DateTime.Today.AddYears(-1).Year, 4, 1); } // If today's month is April through December, then use the current year for "This Fiscal Year" starting point (minimum filter value) by simply using DateTime.Today.Year to set the year, as shown below. if(DateTime.Today.Month >= 4) { _filter.Minimum = new DateTime(DateTime.Today.Year, 4, 1); } } } } public bool KeepFormOpen { get { return false; } } #endregion
필터 적용
FlexGrid에 필터를 적용하려면 Form1.cs로 돌아가 코드의 다음 행을 호출하여 "배송 날짜" 열의 필터를 새 DateFilter 개체로 설정해야 합니다.
c1FlexGrid1.Cols["Ship Date"].Filter = new DateFilter();
이제 응용 프로그램을 실행할 수 있으며 "배송 날짜" 열의 열 필터 편집기를 열면 DateFilterEditor 사용자 컨트롤 클래스에서 체크박스 옵션이 표시됩니다.
필터 중 하나에 대해 체크박스를 선택하면 DateFilterEditor 클래스의 ApplyChanges() 메서드 내부에서 해당하는 항목에 대해 로직이 적용됩니다.
예를 들어 "지난 주" 체크박스를 선택하면 ApplyChanges() 메서드(DateFilterEditor.cs 클래스 내부) 내에서 if(_chkLastWeek.Checked) 블록이 표시된 그리드에 적절한 필터 로직을 적용합니다.
해당 스터디에서 우리가 수행한 작업을 요약하면, 모든 사용자 정의 필터 로직은 개체 다형성을 통해 작동한다는 것입니다.
IC1ColumnFilter 및 IC1ColumnFilterEditor 인터페이스를 구현하면 사용자 정의 DateFilter 및 DateFilterEditor 클래스는 각각 IC1ColumnFilter 및 IC1ColumnFilterEditor 유형을 상속합니다.
FlexGrid 열 필터는 인터페이스 구현을 통해 적용되는 다형성 원칙 덕분에 DateFilter 개체와 마찬가지로 IC1ColumnFilter 유형의 개체로 설정할 수 있습니다.
지금 바로 ComponentOne을 다운로드하여 직접 테스트해 보세요!
댓글목록
등록된 댓글이 없습니다.